Anno 1800 Console Edition
- Ubisoft Blue Byte Mainz - published
- PS5 / XBox Series X|S - C++
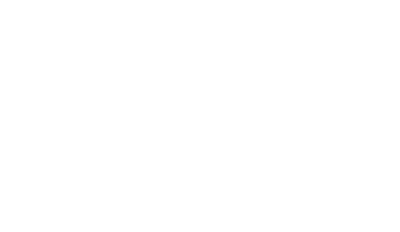
In Anno 1800 you are able to design huge metropolises, manage an emerging economy and protect your creations from others.
To let your cities flourish, you'll have to learn to take the right measures in any situation.
Anno 1800 offers you plenty of opportunities to prove your skills.
Here you'll be able to build huge cities, plan logistic networks, colonize new fertile continents, undertake expeditions around the globe
and dominate your opponents through diplomacy, trade or warfare.
Website: ubisoft.com/de-de/game/anno/1800/console-edition
To let your cities flourish, you'll have to learn to take the right measures in any situation.
Anno 1800 offers you plenty of opportunities to prove your skills.
Here you'll be able to build huge cities, plan logistic networks, colonize new fertile continents, undertake expeditions around the globe
and dominate your opponents through diplomacy, trade or warfare.
My Tasks
- Controller Input
- Build Modes and Build Tools
- Naval Combat
- UI Programming
Screenshots
Videos